In [1]:
import numpy
import matplotlib.pyplot as plt
In [2]:
%matplotlib inline
Infinity normΒΆ
In [3]:
def norm(v, order):
return sum([vi**order for vi in v])**(1/float(order))
In [4]:
v = [1, 2, 3, 2, 1]
norm(v, 10)
Out[4]:
3.010255858106243
In [5]:
plt.plot(range(1, 10), [norm(v, order) for order in range(1, 10)])
Out[5]:
[<matplotlib.lines.Line2D at 0x11b1295c0>]
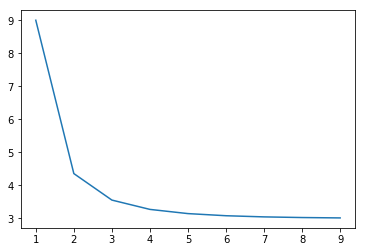
In [6]:
G = numpy.matrix([[5, 4],
[3, 2]])
In [7]:
d = numpy.array([[1], [1]])
In [8]:
G*d
Out[8]:
matrix([[9],
[5]])
In [9]:
def gain(theta):
d = numpy.matrix([[numpy.cos(theta[0])], [numpy.sin(theta[0])]])
return numpy.linalg.norm(G*d)/numpy.linalg.norm(d)
In [10]:
import scipy.optimize
In [11]:
scipy.optimize.minimize(lambda theta: -gain(theta), [1])
Out[11]:
fun: -7.343420458864692
hess_inv: array([[ 0.13636409]])
jac: array([ 5.96046448e-08])
message: 'Optimization terminated successfully.'
nfev: 18
nit: 4
njev: 6
status: 0
success: True
x: array([ 0.65390079])